6장 실습문제 1번 : MyPoint 클래스 작성하기
class MyPoint {
int x, y;
public MyPoint(int x, int y) {
this.x = x;
this.y = y;
}
@Override
public String toString() {
return "Point (" + x + ", " + y + ")";
}
public boolean equals(Object obj) {
MyPoint p = (MyPoint)obj;
if(x==p.x && y==p.y)
return true;
else return false;
}
}
public class Ex6_01 {
public static void main(String[] args) {
MyPoint p = new MyPoint(3, 50);
MyPoint q = new MyPoint(4, 50);
System.out.println(p);
if(p.equals(q))
System.out.println("같은 점");
else
System.out.println("다른 점");
}
}
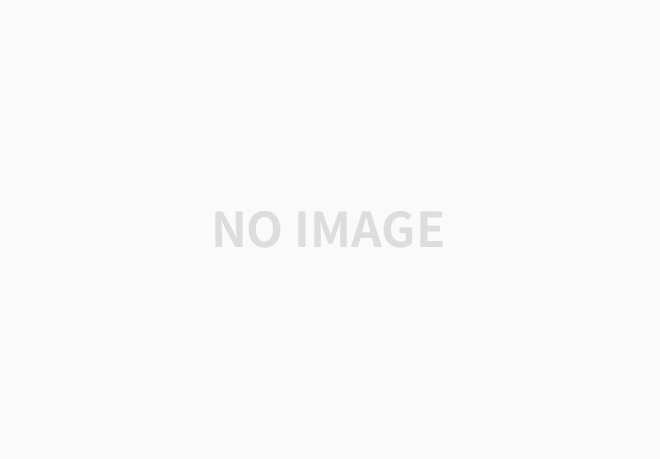
6장 실습문제 2번 : 중심을 나타내는 정수 x, y와 반지름 radius 필드를 가지는 Circle 클래스를 작성한다. 생성자는 3개의 인자(x, y, radius)를 받아 해당 필드를 초기화하고 equals() 메소드는 두 개의 Circle 객체의 중심이 같으면 같은 것으로 판별하도록 한다.
class Circle {
int x, y, radius;
public Circle(int x, int y, int radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
@Override
public String toString() {
return "Circle : (" + x + "," + y + ") radius=" + radius;
}
public boolean equals(Object obj) {
Circle c = (Circle)obj;
if(x==c.x && y==c.y) return true;
else return false;
}
}
public class Ex6_02 {
public static void main(String[] args) {
Circle a = new Circle(2,3,5);
Circle b = new Circle(2,3,30);
System.out.println("원 a : "+a);
System.out.println("원 b : "+b);
if(a.equals(b))
System.out.println("같은 원");
else
System.out.println("서로 다른 원");
}
}
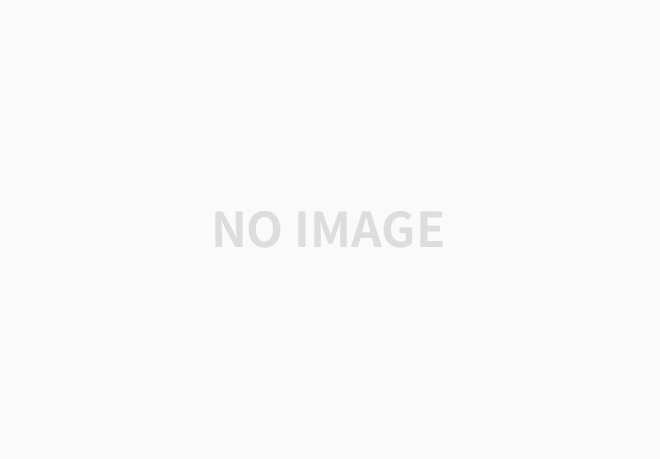
6장 실습문제 3번 : Calc 클래스는 etc 패키지에, MainApp 클래스는 main 패키지로 분리 작성하라.
package etc;
public class Calc {
private int x, y;
public Calc(int x, int y) {
this.x = x;
this.y = y;
}
public int sum() {return x+y;}
}
package main;
import etc.Calc;
public class MainApp {
public static void main(String[] args) {
Calc c = new Calc(10, 20);
System.out.println(c.sum());
}
}
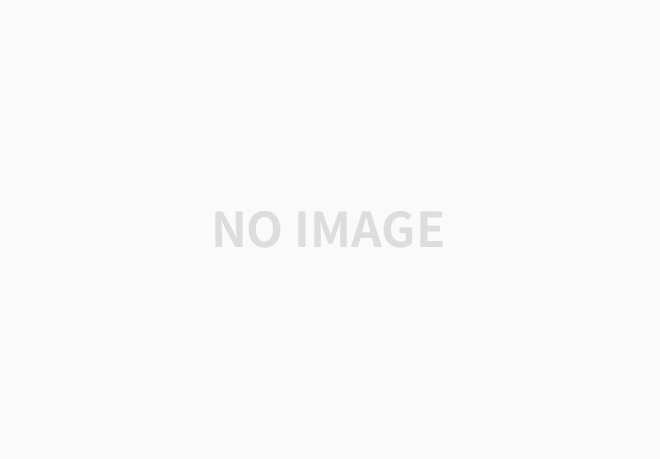
6장 실습문제 4번 : Shape 클래스는 base 패키지에, Circle 클래스는 derived 패키지에, GraphicEditor 클래스는 app 패키지에 분리 작성하라.
package base;
public class Shape {
public void draw() {System.out.println("Shape");}
}
package derived;
import base.Shape;
public class Circle extends Shape {
public void draw() {System.out.println("Circle");}
}
package app;
import base.Shape;
import derived.Circle;
public class GraphicEditor {
public static void main(String[] args) {
Shape shape = new Circle();
shape.draw();
}
}
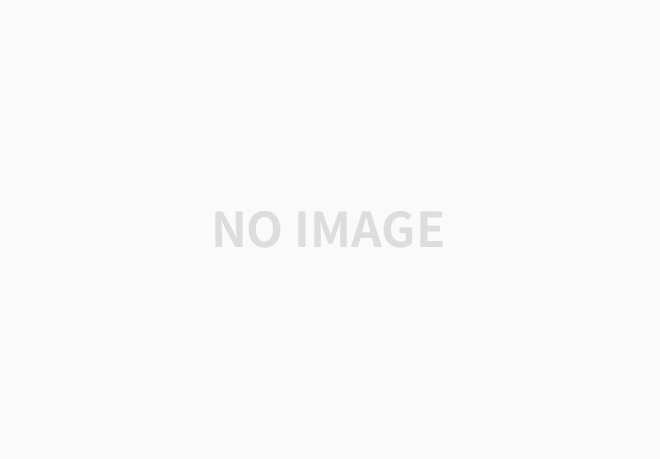
6장 실습문제 5번 : 프로그램을 실행한 현재 시간이 새벽 4시에서 낮 12시 이전이면 "Good Morning"을, 오후 6시 이전이면 "Good Afternoon"을, 밤 10시 이전이면 "Good Evening"을, 그 이후는 "Good Night"을 출력하는 프로그램을 작성하라.
import java.util.*;
public class Ex6_5 {
public static void main(String[] args) {
Calendar now = Calendar.getInstance();
int hour = now.get(Calendar.HOUR_OF_DAY);
int min = now.get(Calendar.MINUTE);
System.out.println("현재 시간은 "+hour+"시 "+min+"분 입니다.");
if(hour>4&&hour<12) System.out.println("Good Morning!");
else if(hour>=12&&hour<18) System.out.println("Good Afternoon!");
else if(hour>=18&&hour<22) System.out.println("Good Evening!");
else System.out.println("Good Night!");
}
}
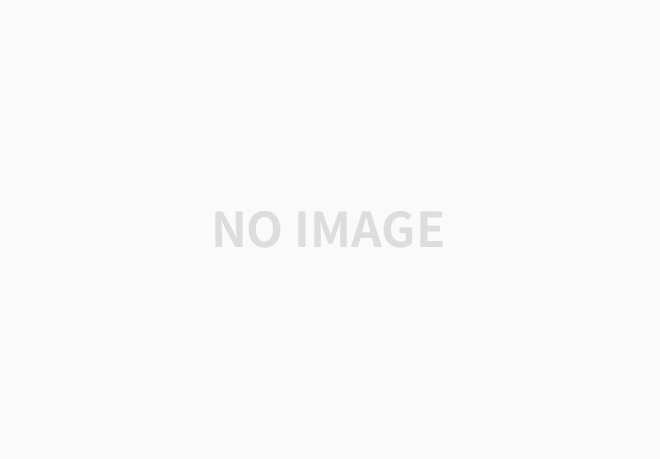
6장 실습문제 6번 : <Enter> 키를 입력하면 현재 초 시간을 보여주고 여기서 10초에 더 근접하도록 다음 <Enter> 키를 입력한 사람이 이기는 게임을 작성하라.
import java.util.*;
public class Ex6_06 {
public static int enter() {
Scanner sc = new Scanner(System.in);
sc.nextLine();
Calendar now = Calendar.getInstance();
return now.get(Calendar.SECOND);
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
//게임에 참여할 사람 수 받기
System.out.println("게임에 참가할 인원은 몇 명입니까? >> ");
System.out.println("(참가인원을 입력하면 바로 게임이 시작됩니다)");
int num = sc.nextInt();
//참여받은 사람만큼 배열 생성
int arr[] = new int[num];
//게임 시작
for(int i=0; i<arr.length; i++) {
Calendar now = Calendar.getInstance();
int sec = now.get(Calendar.SECOND);
System.out.println("=========game start!=========");
System.out.println((i+1)+"번째 참가자님, 현재 시간은 "+sec+"초 입니다.");
int ran = (int)(Math.random()*10+1);
System.out.print("10초 뒤 enter를 누르세요");
int sec1 = enter();
if(sec1 > sec) {
arr[i] = sec1 - sec;
}
else {
arr[i] = 60 - sec + sec1;
}
System.out.println((i+1)+"번째 참가자님의 기록 : "+arr[i]+"초");
}
//10초에 가장 가까이 누른 사람 찾기
int cnt=0;
for(int i=0; i<arr.length; i++) {
if(arr[i]<=10) {
if(10-arr[i] < 10-arr[cnt]) cnt = i;
}
}
System.out.println("10초에 가장 가깝게 누른 사람은 "+(cnt+1)+"번째 참가자입니다.");
}
}
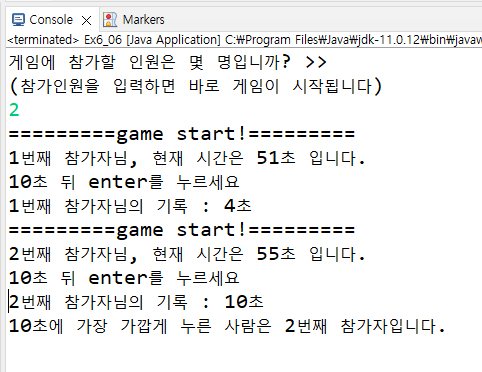
6장 실습문제 7번 (1) : StringTokenizer 클래스를 이용하여 작성하라
Scanner를 이용하여 한 라인을 읽고, 공백으로 분리된 어절이 몇 개 들어 있는지 "그만"을 입력할 때까지 반복하는 프로그램을 작성하라.
import java.util.*;
public class Ex6_07_1 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while(true) {
System.out.println("원하는 문장을 입력하세요.");
System.out.print(">>");
String s = sc.nextLine();
if(s.equals("그만")) {
System.out.println("종료합니다...");
break;
}
StringTokenizer st = new StringTokenizer(s, " ");
int n = st.countTokens();
System.out.println("어절 개수는 : "+n);
}
}
}
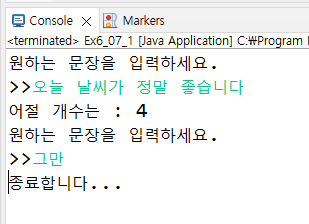
6장 실습문제 7번 (2) : String 클래스의 split() 메소드를 이용하여 작성하라.
Scanner를 이용하여 한 라인을 읽고, 공백으로 분리된 어절이 몇 개 들어 있는지 "그만"을 입력할 때까지 반복하는 프로그램을 작성하라.
import java.util.*;
public class Ex6_07_2 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
while(true) {
System.out.println("원하는 문장을 입력하세요.");
System.out.print(">>");
String s = sc.nextLine();
if(s.equals("그만")) {
System.out.println("종료합니다...");
break;
}
String arr[];
arr = s.split(" ");
System.out.println("어절 개수는 : "+arr.length);
}
}
}
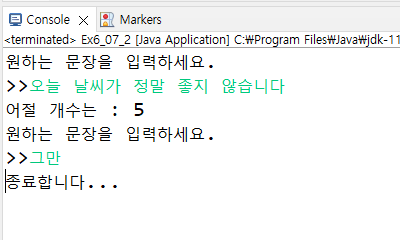
6장 실습문제 8번 : 문자열을 입력받아 한 글자씩 회전시켜 모두 출력하는 프로그램을 작성하라.
import java.util.Scanner;
public class Ex6_08 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("문자열을 입력하세요. 빈칸이 있어도 되고 영어 한글 모두 됩니다.");
String s = sc.nextLine();
for(int i=1; i<=s.length(); i++) {
System.out.print(s.substring(i));
for(int j=0; j<i; j++) {
System.out.print(s.charAt(j));
}
System.out.println();
}
}
}
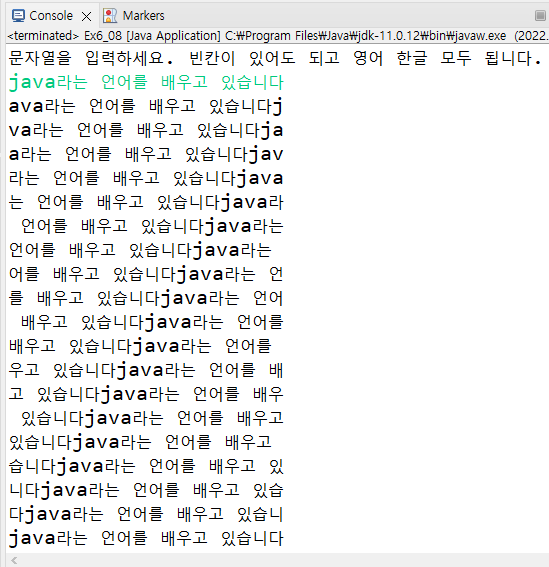
6장 실습문제 9번 : 철수와 컴퓨터의 가위바위보 게임을 만들어보자. 가위, 바위, 보는 각각 1, 2, 3 키이다. 철수가 키를 입력하면, 동시에 프로그램도 Math.Random()을 이용하여 1, 2, 3 중에 한 수를 발생시키고 이것을 컴퓨터가 낸 것으로 한다. 그런 다음 철수와 컴퓨터 중 누가 이겼는지 판별하여 승자를 출력하라.
import java.util.Scanner;
class Player {
private String name;
Player(String name) {
this.name = name;
}
public String getName() {
return name;
}
public int select() {
Scanner sc = new Scanner(System.in);
System.out.print("철수[가위(1), 바위(2), 보(3), 끝내기(4)]>>");
return sc.nextInt();
}
}
class Computer extends Player {
public Computer(String name) {
super(name);
}
public int select() {
return (int)(Math.random() * 3 + 1);
}
}
class Game {
private String[] game = {"가위", "바위", "보"};
Player[] player = new Player[2];
public Game() {
player[0] = new Player("철수");
player[1] = new Computer("컴퓨터");
}
public void run() {
int playerNum, computerNum;
while(true) {
playerNum = player[0].select();
if(playerNum < 1 || playerNum > 4)
System.out.println("잘못 입력했습니다.");
else if(playerNum == 4)
break;
computerNum = player[1].select();
System.out.print(player[0].getName() + "(" + game[playerNum - 1] + ") : ");
System.out.println(player[1].getName() + "(" + game[computerNum - 1] + ")");
if(playerNum == computerNum)
System.out.println("비겼습니다.");
else if((playerNum == 1 && computerNum == 2) || (playerNum == 2 && computerNum == 3) || (playerNum == 3 && computerNum == 1))
System.out.println(player[1].getName() + "가 이겼습니다.");
else if((playerNum == 1 && computerNum == 3) || (playerNum == 2 && computerNum == 1) || (playerNum == 3 && computerNum == 2))
System.out.println(player[0].getName() + "가 이겼습니다.");
}
}
}
public class Ex6_09 {
public static void main(String[] args) {
Game game = new Game();
game.run();
}
}
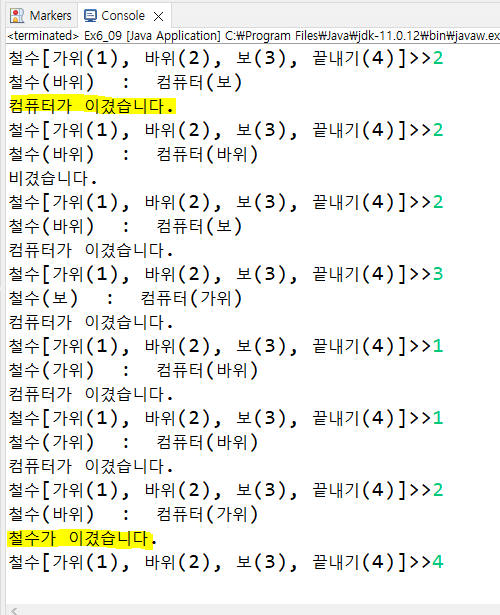
6장 실습문제 10번 : 갬블링 게임을 만들어보자. 두 사람이 게임을 진행한다. 이들의 이름을 키보드로 입력 받으며 각 사람은 Person 클래스로 작성하라. 두 사람은 번갈아 가면서 자기 차례에서 <Enter> 키를 입력하면, 3개의 난수가 발생되고 이 숫자가 모두 같으면 승자가 되고 게임이 끝난다.
import java.util.Scanner;
class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void turn() {
System.out.print("[" + name + "]:<Enter>");
}
public boolean randomNum() {
int num[] = new int[3];
boolean win = true;
for(int i = 0; i < num.length; i++) {
num[i] = (int)(Math.random() * 3 + 1);
System.out.print(num[i] + "\t");
}
for(int i = 0; i < num.length; i++) {
if(!(num[0] == num[i])) {
win = false;
break;
}
}
return win;
}
}
class Game {
Scanner sc = new Scanner(System.in);
Person[] person = new Person[2];
public Game() {
for(int i = 0; i < person.length; i++) {
System.out.print((i+1) + "번째 선수 이름>>");
person[i] = new Person(sc.next());
}
}
public void run() {
while(true) {
for(int i = 0; i < person.length; i++) {
sc.nextLine();
person[i].turn();
sc.nextLine();
if(person[i].randomNum()) {
System.out.print(person[i].getName() + "님이 이겼습니다!");
return;
}
else System.out.print("아쉽네요 다시 도전하세요!");
}
}
}
}
public class Ex6_10 {
public static void main(String[] args) {
Game game = new Game();
game.run();
}
}
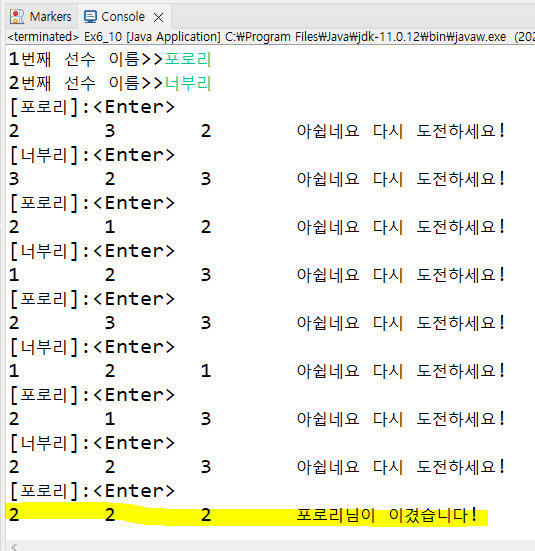
6장 실습문제 11번 : StringBuffer 클래스를 활용하여 문자열을 수정하라.
import java.util.Scanner;
public class Ex6_11 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("====문장 입력====");
System.out.print(">>");
String content = sc.nextLine();
StringBuffer sb = new StringBuffer(content);
while (true) {
System.out.println("명령을 입력하세요 >> ");
System.out.println("명령 형식 : (수정하고 싶은 단어)!(새로운 단어)");
String str = sc.nextLine();
if (str.equals("그만")) {
System.out.println("종료합니다");
break;
}
String[] tokens = str.split("!");
if(tokens.length != 2)
System.out.println("잘못된 명령입니다!");
else {
if(tokens[0].length() == 0 || tokens[1].length() == 0) {
System.out.println("잘못된 명령입니다!");
continue;
}
int index = sb.indexOf(tokens[0]);
if(index == -1) {
System.out.println("찾을 수 없습니다!");
continue;
}
sb.replace(index, index + tokens[0].length(), tokens[1]);
System.out.println(sb.toString());
}
}
}
}
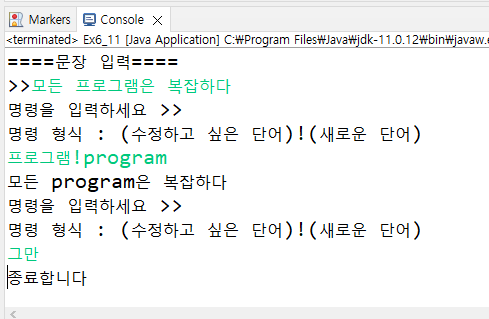
6장 실습문제 12번 : 문제 10의 갬블링 게임을 n명이 하도록 수정하라.
import java.util.Scanner;
class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void turn() {
System.out.print("[" + name + "]:<Enter>");
}
public boolean randomNum() {
int num[] = new int[3];
boolean win = true;
for(int i = 0; i < num.length; i++) {
num[i] = (int)(Math.random() * 3 + 1);
System.out.print(num[i] + "\t");
}
for(int i = 0; i < num.length; i++) {
if(!(num[0] == num[i])) {
win = false;
break;
}
}
return win;
}
}
class Game {
Scanner sc = new Scanner(System.in);
Person[] person;
public Game() {
System.out.print("갬블링 게임에 참여할 선수 숫자>>");
int n = sc.nextInt();
person = new Person[n];
for(int i = 0; i < person.length; i++) {
System.out.print((i+1) + "번째 선수 이름>>");
person[i] = new Person(sc.next());
}
}
public void run() {
while(true) {
for(int i = 0; i < person.length; i++) {
sc.nextLine();
person[i].turn();
sc.nextLine();
if(person[i].randomNum()) {
System.out.print(person[i].getName() + "님이 이겼습니다!");
return;
}
else System.out.print("아쉽네요 다시 도전하세요!");
}
}
}
}
public class Ex6_12 {
public static void main(String[] args) {
Game game = new Game();
game.run();
}
}

'개발자 세릴리 > JAVA' 카테고리의 다른 글
[JAVA] 명품자바프로그래밍 5장 실습 (0) | 2023.03.13 |
---|---|
[JAVA] 명품자바프로그래밍 4장 실습 (0) | 2023.02.28 |
[JAVA] 명품자바프로그래밍 3장 실습 (2) | 2023.02.25 |
[JAVA] 명품자바프로그래밍 2장 실습 (0) | 2023.02.23 |