4장 실습문제 1번 : TV클래스를 작성하라
class TV {
//속성
private String name;
private int year;
private int size;
//생성자
TV(String name, int year, int size) {
this.name = name;
this.year = year;
this.size = size;
}
//메소드
void show() {
System.out.println(name+" 에서 만든 "+year+"년형 "+size+"인치 TV입니다.");
}
}
public class Ex4_1 {
public static void main(String[] args) {
TV tv = new TV("LG", 2022, 75);
tv.show();
}
}
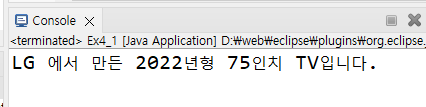
4장 실습문제 2번 : Grade 클래스를 작성하라. 3 과목의 점수를 입력받아 Grade 객체를 생성하고 성적 평균을 출력한다.
//Grade 클래스를 작성해보자.
//수학, 과학, 영어 3개 과목을 입력받아 Grade 객체를 생성하고 출력하는
//main()의 실행예시는 다음과 같다
public class Grade {
//속성
int math;
int science;
int eng;
//생성자
Grade() {}
Grade(int math, int science, int eng){
this.math = math;
this.science = science;
this.eng = eng;
}
//average 메소드
double average(int math, int science, int eng) {
return (math+science+eng)/3.0;
}
//main 메소드
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("수학, 과학, 영어 점수를 순서대로 입력 >>");
int math = sc.nextInt();
int science = sc.nextInt();
int eng = sc.nextInt();
Grade me = new Grade(math,science,eng);
System.out.println("평균은 "+me.average(math,science,eng));
}
}
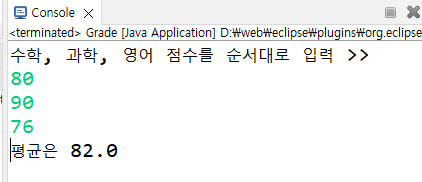
4장 실습문제 3번 : 노래 한 곡을 나타내는 Song 클래스 작성
//노래 한곡을 위한 Song 클래스를 작성하라.
public class Song {
//속성
String title; //노래 제목
String artist; //가수
int year; //노래가 발표된 연도
String country; //국적
//생성자 2개
Song(){}
Song(int year, String country, String artist, String title){
this.year = year;
this.country = country;
this.artist = artist;
this.title = title;
}
//메소드
void show() {
System.out.print(this.year+", ");
System.out.print(this.country+"국적, ");
System.out.print(this.artist+", ");
System.out.println(this.title);
}
public static void main(String[] args) {
//1978, 스웨덴 국적의 ABBA, Dancing Queen을 Song 객체로 생성하고
//show()를 이용하여 노래의 정보를 출력한다.
//출력결과
//1978년, 스웨덴 국적, ABBA, Dancing Queen
Song song1 = new Song(1978,"스웨덴","ABBA","Dancing Queen");
song1.show();
}
}

4장 실습문제 4번 : 직사각형을 표현하는 Rectangle 클래스 작성하라
public class Rectangle {
private int x, y, width, height;
public Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
int square() {
return width*height;
}
void show() {
System.out.println("(" + x + "," + y + ")에서 크기가 " + width + " x " + height + " 인 사각형");
}
boolean contains(Rectangle r) {
if((x+width)>(r.x +r.width) && (y+width)>(r.y+r.width)
&& (x+height)>(r.y+r.height) && (y+height)>(r.y+r.height)) {
return true;
}
else return false;
}
public static void main(String[] args) {
Rectangle r = new Rectangle(2, 2, 8, 7);
Rectangle s = new Rectangle(5, 5, 6, 6);
Rectangle t = new Rectangle(1, 1, 10, 10);
System.out.print("사각형 r은 ");
r.show();
System.out.println("s의 면적은 "+s.square());
if(t.contains(r))
System.out.println("t는 r을 포함합니다.");
if(t.contains(s))
System.out.println("t는 s를 포함합니다.");
}
}
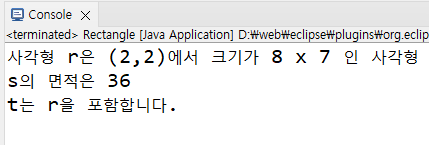
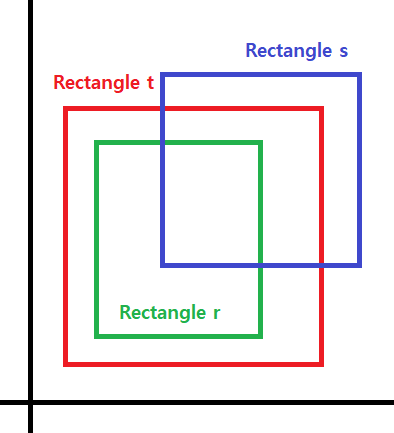
4장 실습문제 5번 : Circle 클래스와 CircleManager 클래스를 완성하라
class Circle {
private double x,y;
private int radius;
public Circle(double x, double y, int radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
public void show() {
System.out.println("(x,y) >> (" + x + "," + y + ")"+" radius >> "+radius);
}
}
public class CircleManager {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Circle c[] = new Circle[3];
for(int i=0; i<c.length; i++) {
System.out.println("x, y, radius >> ");
double x = sc.nextDouble();
double y = sc.nextDouble();
int radius = sc.nextInt();
c[i] = new Circle(x, y, radius);
}
for (int i=0; i<c.length; i++) {
c[i].show();
}
}
}
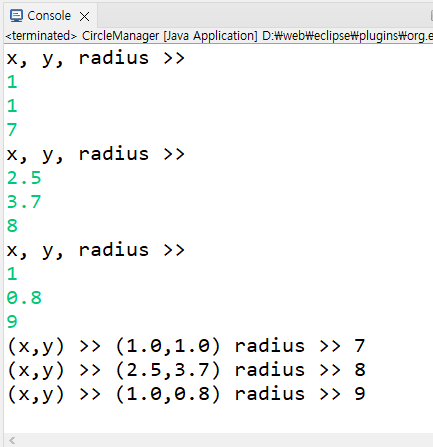
4장 실습문제 6번 : 5번 문제의 Circle 클래스와 CircleManager 클래스를 수정하여 가장 면적이 큰 원을 출력하자.
class Circle {
private double x,y;
private int radius;
public Circle(double x, double y, int radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
public void show() {
System.out.println("(x,y) >> (" + x + "," + y + ")"+" radius >> "+radius);
}
//private radius 값을 받아올 get 함수 생성
public int getRadius() {
return radius;
}
}
public class CircleManager {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Circle c[] = new Circle[3];
int max = 0;
for(int i=0; i<c.length; i++) {
System.out.println("x, y, radius >> ");
double x = sc.nextDouble();
double y = sc.nextDouble();
int radius = sc.nextInt();
c[i] = new Circle(x, y, radius);
}
for(int i=0; i<c.length; i++) {
if(c[i].getRadius() > c[max].getRadius()) {
max = i;
}
}
System.out.println("가장 면적이 큰 원은 ");
c[max].show();
}
}
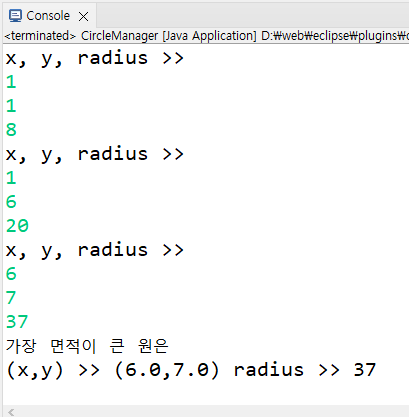
4장 실습문제 7번 : 한 달의 할 일을 표현하는 MonthSchedule 클래스를 작성하라. MonthSchedule 클래스에는 Day 객체 배열과 적절한 필드, 메소드를 작성하고 입력, 보기, 끝내기 등의 3개의 기능을 작성하라.
class Day {
private String work;
public void set(String work) {
this.work = work;
}
public String get() {
return work;
}
public void show() {
if(work==null) {
System.out.println("없습니다.");
}
else {
System.out.println(work+"입니다.");
}
}
}
class MonthSchedule {
Scanner sc = new Scanner(System.in);
private int d;
private Day day[];
public MonthSchedule(int d) {
this.d = d;
day = new Day[d];
for(int i=0; i<day.length; i++) {
day[i] = new Day();
}
}
public void run() {
System.out.println("4월달 스케쥴 관리 프로그램 >> ");
while(true) {
System.out.println("할일 :: 1.입력 2.보기 3.끝내기");
int n = sc.nextInt();
if(n==1) input();
else if(n==2) view();
else if(n==3) {
finish();
break;
}
}
}
public void input() {
System.out.println("날짜(1~30) >> ");
int a = sc.nextInt();
System.out.println("할일(빈칸없이 입력) >> ");
String work = sc.next();
day[a-1].set(work);
System.out.println();
}
public void view() {
System.out.println("날짜(1~30) >> ");
int a = sc.nextInt();
System.out.print(a+"일의 할 일은 ");
day[a-1].show();
System.out.println();
}
public void finish() {
System.out.println("시스템을 종료합니다...");
System.exit(0);
sc.close();
}
}
public class Ex4_7 {
public static void main(String[] args) {
MonthSchedule April = new MonthSchedule(30);
April.run();
}
}
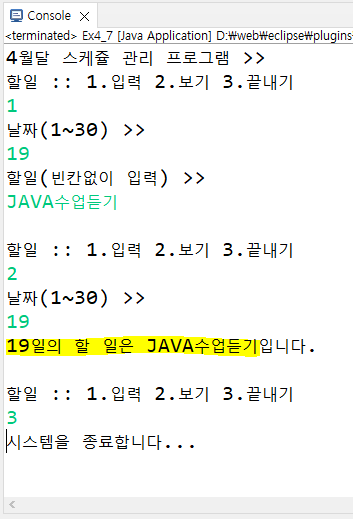
4장 실습문제 8번 : 이름, 전화번호 필드와 생성자 등을 가진 Phone 클래스를 작성하고, 작동시키는 PhoneBook 클래스를 작성하라.
//이름(name), 전화번호(tel)필드와 생성자 등을 가진 Phone클래스를 작성하라
//실행예시처럼 이름으로 전화번호를 검색하라
//인원수 >> 3
//이름과 전화번호 >> 김철수 000-0000
//이름과 전화번호 >> 홍길동 012-0123
//이름과 전화번호 >> 박영희 123-1234
//저장되었습니다.
//검색할 이름 >>> 홍길동
//012-0123
//검색할 이름 >>> 나길순
//검색할 내용이 없습니다.
class Phone{
//속성
private String name;
private String tel;
//생성자
Phone(){}
public Phone(String name, String tel) {
this.name = name;
this.tel = tel;
}
//getters & setters
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
//Source-toString : 자동으로 return값을 만들어 생성해준다
@Override
public String toString() {
return "Phone [name=" + name + ", tel=" + tel + "]";
}
}
//인원수를 받고, 인원수 만큼 배열을 만들어 초기화 합니다.
//인원수 만큼 이름과 전화번호를 입력받아 생성하고 값을 초기화합니다.
//입력이 끝나면 "저장되었습니다" 라고 출력합니다. 그 후,
//검색할 이름을 입력 받습니다.
//검색어가 있다면 전화번호를 출력하고, 없다면 "없습니다" 라고 출력합니다.
//검색은 사용자가 검색할 이름에 "그만" 이라고 입력할 때까지 반복합니다.
public class PhoneBook {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("몇 명의 전화번호부를 생성할까요?>>");
int n = sc.nextInt();
Phone ph[] = new Phone[n];
//전화번호부 생성
for(int i=0; i<ph.length; i++) {
System.out.println("이름과 전화번호를 입력하세요 >>");
String name = sc.next();
String tel = sc.next();
ph[i] = new Phone(name,tel);
}
System.out.println("저장되었습니다.");
//for-each문으로 배열 ph에 저장된 것을 toString에 넣을 수 있다
//for(Phone p : ph) p.toString();
//검색으로 필요한 정보 출력
while(true) {
System.out.println("검색할 이름을 입력하세요 >>");
String search = sc.next();
int count = 0;
for(int i=0; i<ph.length; i++) {
if (search.equals("그만")) {
System.out.println("시스템을 종료합니다...");
//시스템 완전 종료
System.exit(0);
}
else if (search.equals(ph[i].getName())) {
System.out.println(ph[i].getTel());
count++;
}
}
if(count==0) System.out.println("정보가 없습니다.");
}
}
}
4장 실습문제 9번 : 배열을 합치고, print하는 2개의 static 가진 ArrayUtil 클래스를 완성하라.
class ArrayUtil {
public static int [] concat(int[]a, int[]b) {
int [] arr = new int[a.length+b.length];
for(int i=0; i<a.length; i++) {
arr[i] = a[i];
}
for(int i=a.length; i<a.length+b.length; i++) {
arr[i] = b[i-a.length];
}
return arr;
}
public static void print(int[] a) {
System.out.print("[");
for(int x : a) {System.out.print(x+" ");}
System.out.println("]");
}
public static void main(String[] args) {
int[] array1 = {1, 5, 7, 9};
int[] array2 = {3, 6, -1, 100, 77};
int[] array3 = ArrayUtil.concat(array1, array2);
ArrayUtil.print(array3);
}
}
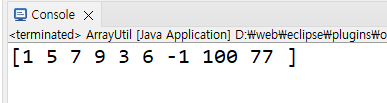
4장 실습문제 10번 : Dictonary 클래스의 kor2Eng() 메소드와 DicApp 클래스를 작성하라.
class Dictionary {
private static String[] kor = {"사랑","아기","돈","미래","희망"};
private static String[] eng = {"love","baby","money","future","hope"};
public static String kor2Eng(String word) {
for(int i=0; i<kor.length; i++) {
if(word.equals(kor[i])) {
return kor[i]+"는(은) "+eng[i];
}
else continue;
}
return word+"는(은) 사전에 없습니다.";
}
}
public class Ex4_10 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("한영 단어 검색 프로그램입니다.");
while(true) {
System.out.println("검색할 한국어 입력 >> ");
String input = sc.next();
if(input.equals("그만")) break;
System.out.println(Dictionary.kor2Eng(input));
}
}
}
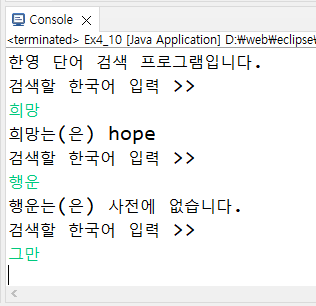
4장 실습문제 11번 : 사칙연산을 수행하는 클래스를 만들어라. main() 메소드에서는 두 정수와 연산자를 입력받고 Add, Sub, Mul, Div 중에서 이 연산을 실행할 수 있는 객체를 생성하고 각 클래스의 메소드를 호출하여 결과를 출력하도록 작성하라.
//덧셈
class Add {
int a,b;
void setValue(int a, int b) {
this.a = a;
this.b = b;
}
int calculate() {
return a+b;
}
}
//뺄셈
class Sub {
int a,b;
void setValue(int a, int b) {
this.a = a;
this.b = b;
}
int calculate() {
return a-b;
}
}
//곱셈
class Mul {
int a,b;
void setValue(int a, int b) {
this.a = a;
this.b = b;
}
int calculate() {
return a*b;
}
}
//나눗셈
class Div {
int a,b;
void setValue(int a, int b) {
this.a = a;
this.b = b;
}
int calculate() {
return a/b;
}
}
public class Ex4_11 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("두 정수 입력 >> ");
int a = sc.nextInt();
int b = sc.nextInt();
System.out.println("연산자 선택 >> 1.덧셈 2.뺄셈 3.곱셈 4.나눗셈");
int n = sc.nextInt();
switch(n) {
case 1:
Add add = new Add();
add.setValue(a, b);
System.out.println(add.calculate());
break;
case 2:
Sub sub = new Sub();
sub.setValue(a, b);
System.out.println(sub.calculate());
break;
case 3:
Mul mul = new Mul();
mul.setValue(a, b);
System.out.println(mul.calculate());
break;
case 4:
try {
Div div = new Div();
div.setValue(a, b);
System.out.println(div.calculate());
} catch (ArithmeticException e) {
if(b==0)
System.out.println("0으로 나눌 수 없습니다.");
}
break;
default:
System.out.println("다시 선택해 주세요");
}
}
}
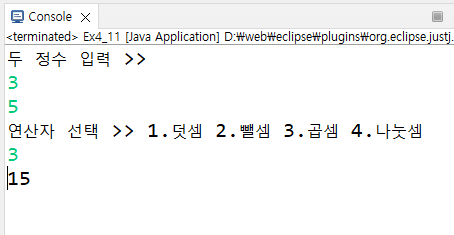
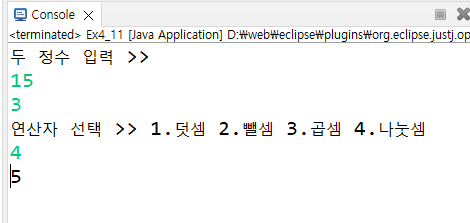
4장 실습문제 12번 : 간단한 콘서트 예약 시스템을 만들어보자.
import java.util.Scanner;
class App {
Scanner sc = new Scanner(System.in);
//속성값
private int seatClass;
private int seatNum;
private String name;
//좌석 클래스=배열 생성
private String seatS[] = new String[10];
private String seatA[] = new String[10];
private String seatB[] = new String[10];
//좌석 초기 세팅
public App() {
for (int i = 0; i < 10; i++) {
seatS[i] = "***";
seatA[i] = "***";
seatB[i] = "***";
}
}
//한 줄의 좌석 전체 보는 메서드
public void viewLine(int seatClass) {
this.seatClass = seatClass;
if(seatClass==1) {
System.out.print("S>> ");
for(String s : seatS) {
System.out.print(s + " ");
}
}
else if (seatClass==2) {
System.out.print("A>> ");
for(String a : seatA) {
System.out.print(a + " ");
}
}
else if (seatClass==3) {
System.out.print("B>> ");
for(String b : seatB) {
System.out.print(b + " ");
}
}
}
//1.예약시스템
public void book() {
Scanner sc = new Scanner(System.in);
System.out.println("좌석구분 S(1), A(2), B(3)>>>");
int seatClass = sc.nextInt();
while (true) {
//S클래스 선택
if (seatClass == 1) {
viewLine(seatClass);
System.out.println();
System.out.println("이름 >> ");
String name = sc.next();
System.out.println("좌석번호 >> ");
int seatNum = sc.nextInt();
if (seatNum > 10 || seatNum < 1) {
System.out.println("없는 좌석 번호입니다. 다시 입력해주세요!");
continue;
}
//사용자가 고른 자리에 이름을 넣음
seatS[seatNum - 1] = name;
}
//A클래스 선택
else if (seatClass == 2) {
viewLine(seatClass);
System.out.println();
System.out.println("이름 >> ");
String name = sc.next();
System.out.println("좌석번호 >> ");
int seatNum = sc.nextInt();
if (seatNum > 10 || seatNum < 1) {
System.out.println("없는 좌석 번호입니다. 다시 입력해주세요!");
continue;
}
//사용자가 고른 자리에 이름을 넣음
seatA[seatNum - 1] = name;
}
//B클래스 선택
else if (seatClass == 3) {
viewLine(seatClass);
System.out.println();
System.out.println("이름 >> ");
String name = sc.next();
System.out.println("좌석번호 >> ");
int seatNum = sc.nextInt();
if (seatNum > 10 || seatNum < 1) {
System.out.println("없는 좌석 번호입니다. 다시 입력해주세요!");
continue;
}
//사용자가 고른 자리에 이름을 넣음
seatB[seatNum - 1] = name;
} else {
System.out.println("잘못 입력했습니다. 다시 시도해주세요.");
continue;
}
System.out.println("---예약이 완료되었습니다---");
break;
}
}
//2.조회시스템
public void viewAll() {
System.out.println(" [ 무대 ]");
viewLine(1); System.out.println();
viewLine(2); System.out.println();
viewLine(3); System.out.println();
System.out.println("---조회를 완료하였습니다---");
}
//3.취소시스템
public void cancel() {
Scanner sc = new Scanner(System.in);
System.out.println("좌석구분 S(1), A(2), B(3)>>>");
int seatClass = sc.nextInt();
while(true) {
int i = 0;
//S클래스 선택
if (seatClass == 1) {
viewLine(seatClass);
System.out.println();
System.out.println("이름 >> ");
String name = sc.next();
for (i = 0; i < seatS.length; i++)
if (seatS[i].equals(name)) { // 입력한 이름과 같은 배열의 좌석을 공석으로 만든다.
seatS[i] = "***";
break;
}
if (i == 10) { // 없는 이름을 입력했을 때
System.out.println("없는 이름입니다. 다시 시도해주세요.");
break;
}
}
//A클래스 선택
else if (seatClass == 2) {
viewLine(seatClass);
System.out.println();
System.out.println("이름 >> ");
String name = sc.next();
for (i = 0; i < seatA.length; i++)
if (seatA[i].equals(name)) { // 입력한 이름과 같은 배열의 좌석을 공석으로 만든다.
seatA[i] = "***";
break;
}
if (i == 10) { // 없는 이름을 입력했을 때
System.out.println("없는 이름입니다. 다시 시도해주세요.");
break;
}
}
//B클래스 선택
else if (seatClass == 3) {
viewLine(seatClass);
System.out.println();
System.out.println("이름 >> ");
String name = sc.next();
for (i = 0; i < seatB.length; i++)
if (seatB[i].equals(name)) { // 입력한 이름과 같은 배열의 좌석을 공석으로 만든다.
seatB[i] = "***";
break;
}
if (i == 10) { // 없는 이름을 입력했을 때
System.out.println("없는 이름입니다. 다시 시도해주세요.");
break;
}
}
else {
System.out.println("잘못 입력했습니다. 다시 입력해주세요.");
continue;
}
System.out.println("---취소를 완료했습니다---");
break;
}
}
}
public class Ex4_12 {
//콘서트 예약 시스템 메인 화면
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥");
System.out.println("♥♥♥♥♥♥ 콘서트 예약 시스템입니다 ♥♥♥♥♥♥");
System.out.println("♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥♥");
App app = new App();
while (true) {
System.out.println("♥♥♥♥♥♥♥ 메뉴를 선택하세요 ♥♥♥♥♥♥♥");
System.out.println("1.예약하기 2.조회하기 3.예약취소 4.끝내기");
int input = sc.nextInt();
switch(input) {
//예약
case 1:
app.book();
break;
//조회
case 2:
app.viewAll();
break;
//취소
case 3:
app.cancel();
break;
//끝내기
case 4:
System.out.println("시스템을 종료합니다...");
System.exit(0);
break;
default:
System.out.println("메뉴를 다시 선택해 주세요");
}
}
}
}
조회하기
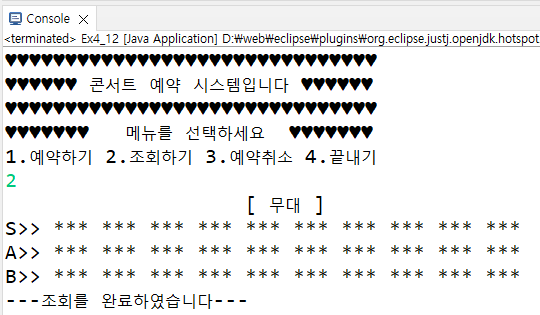
예약하기
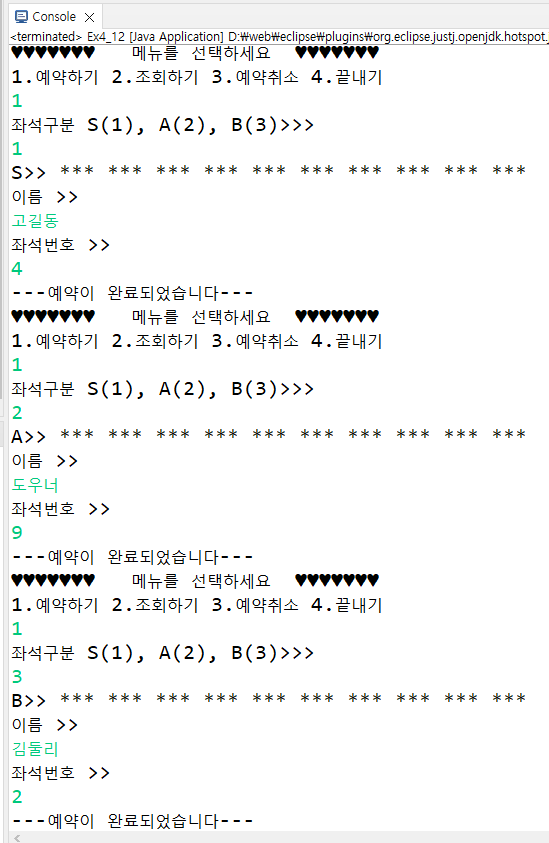
다시 조회하기
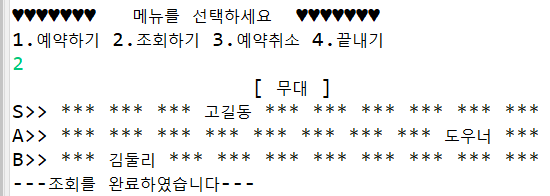
예약취소하기
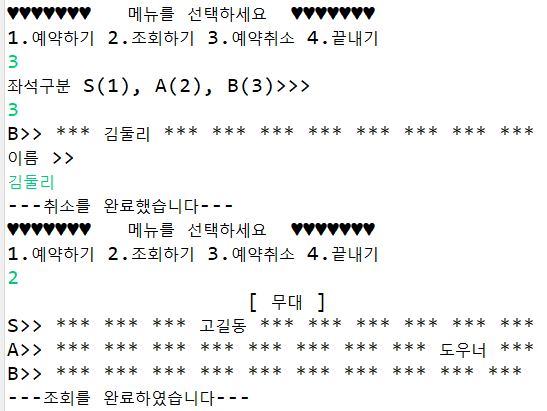
종료하기
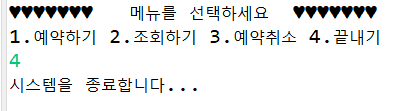
'개발자 세릴리 > JAVA' 카테고리의 다른 글
[JAVA] 명품자바프로그래밍 6장 실습 (0) | 2023.03.16 |
---|---|
[JAVA] 명품자바프로그래밍 5장 실습 (0) | 2023.03.13 |
[JAVA] 명품자바프로그래밍 3장 실습 (2) | 2023.02.25 |
[JAVA] 명품자바프로그래밍 2장 실습 (0) | 2023.02.23 |