2장 실습문제 1번 : 원을 달러로 변환하는 프로그램
//원화를 입력받아 달러로 바꾸어 출력하기
public class Ex2_1 {
public static void main(String[] args) {
final double rate = 1100.0;
System.out.println("원화를 입력하세요(단위 원) >>");
Scanner sc = new Scanner(System.in);
int won = sc.nextInt();
double dollar = won/rate;
System.out.println(won + "원은 $" + dollar + "입니다.");
sc.close();
}
}
2장 실습문제 2번 : 2자리 정수를 입력받고 십의 자리와 일의 자리 비교하기
//스캐너로 10~99 사이의 숫자를 입력받아,
//십의 자리와 일의 자리가 같은지 판별하는 프로그램
public class Ex2_2 {
public static void main(String[] args) {
int a, b, c;
System.out.println("두자리의 정수입력(10~99) >>");
Scanner sc = new Scanner(System.in);
a = sc.nextInt();
b = a/10;
c = a%10;
if (b==c)
System.out.println("10의 자리와 1의 자리가 같습니다.");
else
System.out.println("10의 자리와 1의 자리가 같지 않습니다.");
sc.close();
}
}
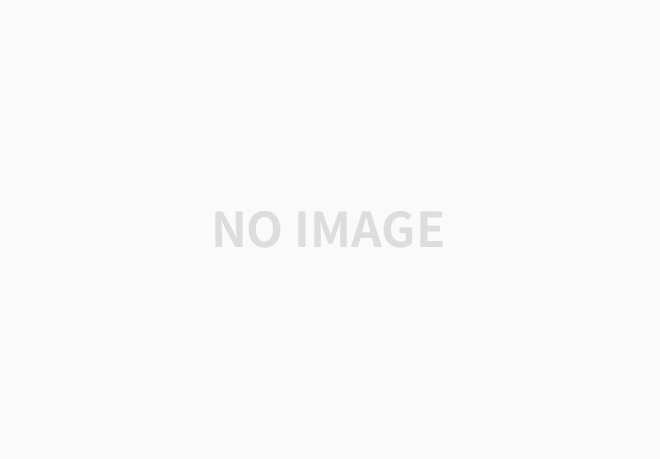
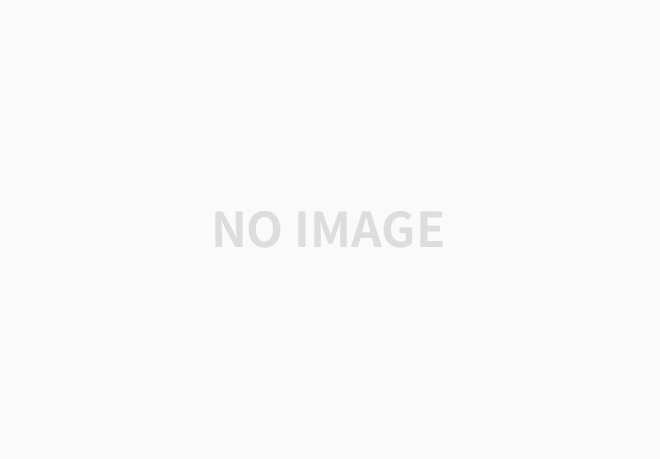
2장 실습문제 3번 : 돈의 액수를 입력받아 화폐단위 별 변환하는 프로그램
public class Ex2_3 {
//돈의 액수를 입력받아 화폐단위 별로 변환하는 프로그램
public static void main(String[] args) {
final int 오만원 = 50000;
final int 만원 = 10000;
final int 오천원 = 5000;
final int 천원 = 1000;
final int 오백원 = 500;
final int 백원 = 100;
final int 오십원 = 50;
final int 십원 = 10;
Scanner sc = new Scanner(System.in);
System.out.println("금액을 입력하세요 >> ");
int money = sc.nextInt();
int a;
a = money/오만원;
money = money%오만원;
if(a>0) {
System.out.println("오만원권 "+a+"매");
}
a = money/만원;
money = money%만원;
if(a>0) {
System.out.println("만원권 "+a+"매");
}
a = money/오천원;
money = money%오천원;
if(a>0) {
System.out.println("오천원권 "+a+"매");
}
a = money/천원;
money = money%천원;
if(a>0) {
System.out.println("천원권 "+a+"매");
}
a = money/오백원;
money = money%오백원;
if(a>0) {
System.out.println("오백원 "+a+"개");
}
a = money/백원;
money = money%백원;
if(a>0) {
System.out.println("백원 "+a+"개");
}
a = money/오십원;
money = money%오십원;
if(a>0) {
System.out.println("오십원 "+a+"개");
}
a = money/십원;
money = money%백원;
if(a>0) {
System.out.println("십원 "+a+"개");
}
}
}
*static 클래스 안에서는 static 선언이 필요 없지만, 클래스 밖에서는 static 선언을 해야 다른 클래스에서 쓸 수가 있다.
*static - 정적인 객체
*instance 변수 - 동적인 객체
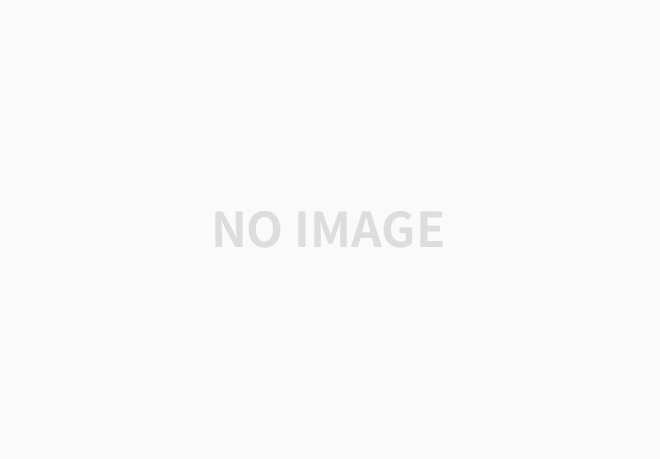
2장 실습문제 4번 : 정수 3개 입력받아 중간 크기의 수 출력하는 프로그램
public class Ex2_4 {
//정수 3개 입력받아 중간 크기의 수 출력하는 프로그램
public static void main(String[] args) {
int a,b,c,temp;
System.out.println("정수 3개 입력하세요 >> ");
Scanner sc = new Scanner(System.in);
a = sc.nextInt();
b = sc.nextInt();
c = sc.nextInt();
if(a>b&&a<c || a>c&&a<b)
temp=a;
else if(b>c&&b<a || b>a&&b<c)
temp=b;
else
temp=c;
System.out.println("중간 값은 "+temp+"입니다.");
}
}
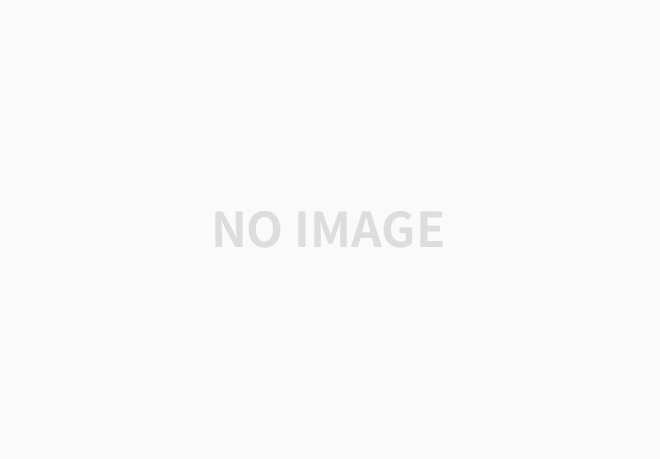
2장 실습문제 5번 : 삼각형 변의 길이 정수 3개를 입력받고 삼각형을 만들 수 있는지 판별하는 프로그램
public class Ex2_5 {
//삼각형 변의 길이 정수 3개를 입력받고
//삼각형을 만들 수 있는지 판별하는 프로그램
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.println("삼각형의 세 변을 입력하시오 >> ");
int a = sc.nextInt();
int b = sc.nextInt();
int c = sc.nextInt();
if((a+b)<=c || (a+c)<=b || (b+c)<=a)
System.out.println("삼각형을 만들 수 없습니다.");
else
System.out.println("삼각형을 만들 수 있습니다.");
sc.close();
}
}
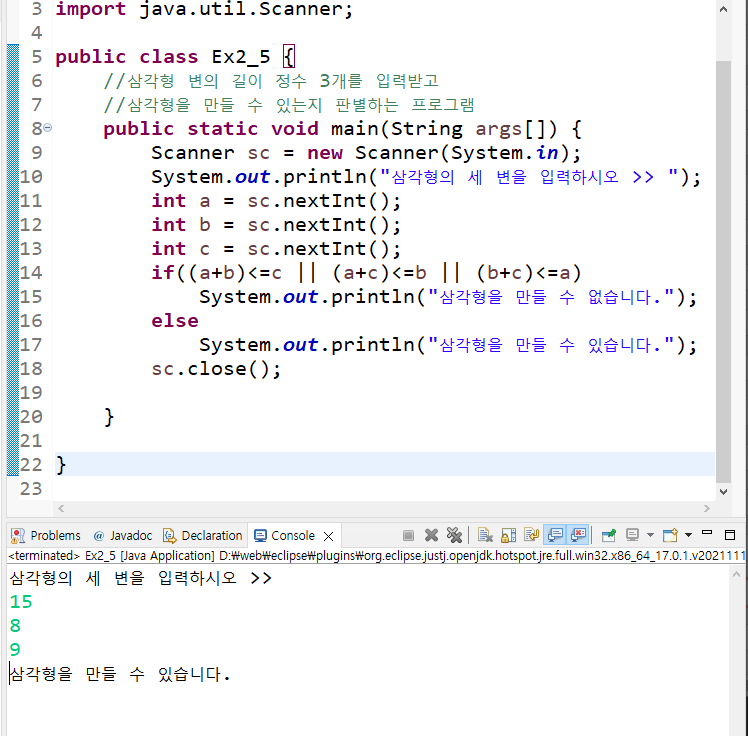
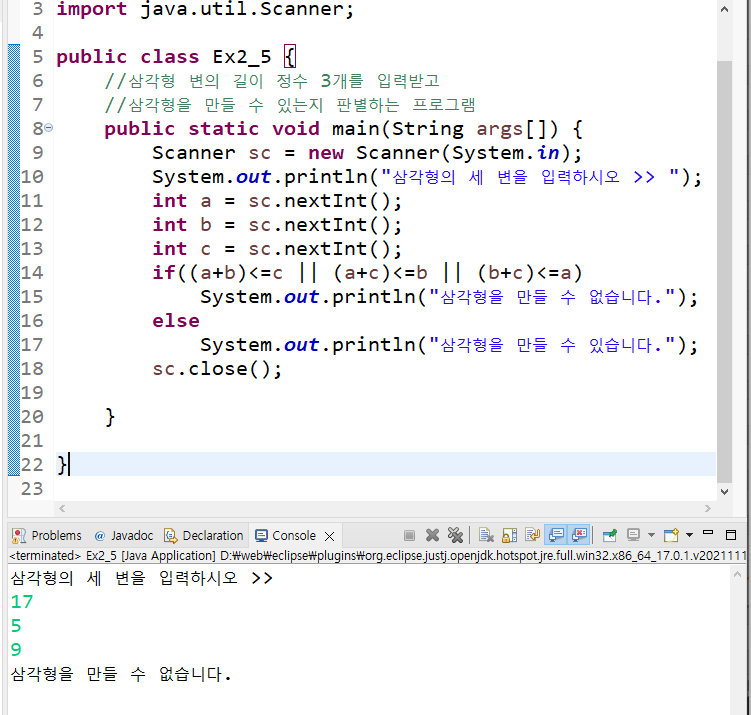
2장 실습문제 6번 : 1~99까지의 정수를 입력 받아 3,6,9 중 하나가 있는 경우는 "박수짝", 두 개 있는 경우는 "박수짝짝" 출력
public class Ex2_6 {
//1~99까지의 정수를 입력 받아
//3,6,9 중 하나가 있는 경우는 "박수짝"
//두 개 있는 경우는 "박수짝짝" 출력
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("1~99 사이의 숫자를 입력하세요 >> ");
int a = sc.nextInt();
int temp1 = a/10;
int temp2 = a%10;
if(temp2==3 || temp2==6 || temp2==9) {
System.out.print("박수짝");
if(temp1==3 || temp1==6 || temp1==9)
System.out.print("짝");
}
else if(temp1==3 || temp1==6 || temp1==9)
System.out.print("박수짝");
sc.close();
}
}
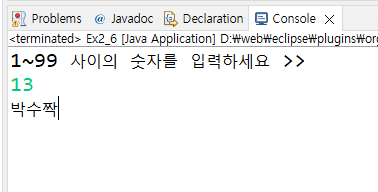
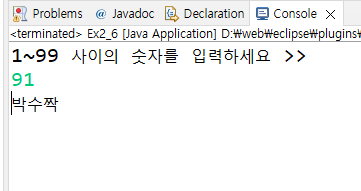
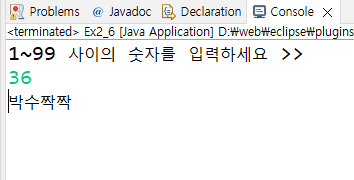
++다른 방법
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("1~99 사이의 숫자를 입력하세요 >>");
int a = sc.nextInt();
int count = 0;
if(a>=1 && a<=99) {
int temp1 = a/10;
int temp2 = a%10;
if (temp1!=0 && temp1%3==0) count++; //temp1!=0은 0이 아닌 수라는 뜻
if (temp2!=0 && temp2%3==0) count++;
if(count == 1) System.out.println("박수짝");
else if(count == 2) System.out.println("박수짝짝");
else System.out.println("박수없어!");
}
sc.close();
}
2장 실습문제 7번 : (100,100)과 (200,200)의 사각형이 있을 때, x,y값을 입력받아 직사각형 안에 있는지 판별하는 프로그램
public class Ex2_7 {
//(100,100)과 (200,200)의 사각형이 있을 때, x,y값을 입력받아
//직사각형 안에 있는지 판별하는 프로그램
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("점 (x,y)의 좌표를 입력하시오 >> ");
int x = sc.nextInt();
int y = sc.nextInt();
if (x>=100 && x<=200 && y>=100 && y<=200)
System.out.println("("+x+","+y+")는 사각형 안에 있습니다.");
else
System.out.println("("+x+","+y+")는 사각형 안에 없습니다.");
}
}
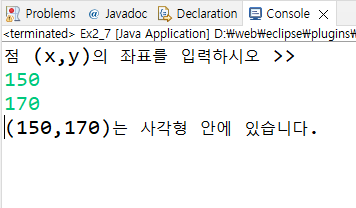
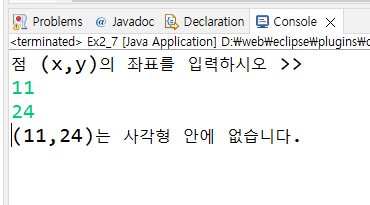
2장 실습문제 8번 : 키보드로부터 직사각형을 구성하는 두 점 (x1,y1), (x2,y2)를 입력받아 (100,100), (200,200)의 두 점으로 이루어진 직사각형과 충돌하는지 판별하는 프로그램
public class Ex2_8 {
//키보드로부터 직사각형을 구성하는 두 점 (x1,y1), (x2,y2)를 입력받아
//(100,100), (200,200)의 두 점으로 이루어진 직사각형과
//충돌하는지 판별하는 프로그램
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("직사각형을 만드는 두 좌표를 입력 하시오 >> ");
System.out.println("(x1,y1) >> ");
int x1 = sc.nextInt();
int y1 = sc.nextInt();
System.out.println("(x2,y2) >> ");
int x2 = sc.nextInt();
int y2 = sc.nextInt();
if (x1>=100 && x1<=200 && y1>=100 && y1<=200)
System.out.println("충돌합니다.");
else if (x2>=100 && x2<=200 && y2>=100 && y2<=200)
System.out.println("충돌합니다.");
else
System.out.println("충돌하지 않습니다.");
sc.close();
}
}
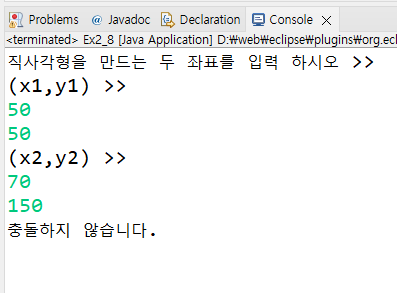
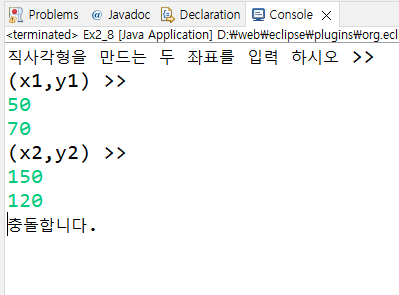
2장 실습문제 9번 : 원의 중심을 나타내는 한 점과 반지름을 실수값으로 입력받고, 실수 값으로 다른 점 (x,y)를 입력받아 이 점이 원의 내부에 있는지 판별하기
public class Ex2_9 {
//원의 중심을 나타내는 한 점과 반지름을 실수값으로 입력받고,
//실수 값으로 다른 점 (x,y)를 입력받아 이 점이 원의 내부에 있는지 판별하기
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("원의 중심 좌표를 입력하시오 (x,y) >> ");
double x = scanner.nextDouble();
double y = scanner.nextDouble();
System.out.println("원의 반지름을 입력하시오 >> ");
double radius = scanner.nextDouble();
System.out.print("확인할 좌표를 입력하시오 (x2,y2) >> ");
double x2 = scanner.nextDouble();
double y2 = scanner.nextDouble();
double distance = Math.sqrt((x-x2)*(x-x2) + (y-y2)*(y-y2));
if(distance < radius)
System.out.print("점 (" + x2 + "," + y2 + ")는 원 내부에 있습니다.");
else
System.out.print("점 (" + x2 + "," + y2 + ")는 원 외부에 있습니다.");
scanner.close();
}
}
*Math.sqrt()는 제곱근을 반환하는 함수
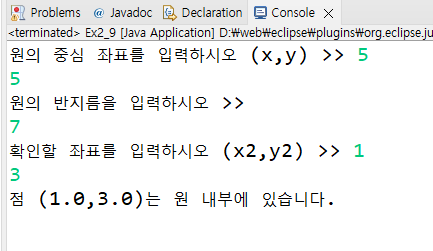
2장 실습문제 10번 : 두 개의 원에 각각의 원의 중심인 한 점과 반지름을 입력받고, 서로 겹치는지 판단하기
public class Ex2_10 {
//두 개의 원에 각각의 원의 중심인 한 점과 반지름을 입력받고,
//서로 겹치는지 판단하기
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("첫 번째 원의 중심을 입력하시오(x1,y1)>> ");
Double x1 = sc.nextDouble();
Double y1 = sc.nextDouble();
System.out.println("첫 번째 원의 반지름을 입력하시오 >> ");
Double radius1 = sc.nextDouble();
System.out.println("두 번째 원의 중심을 입력하시오(x2,y2)>> ");
Double x2 = sc.nextDouble();
Double y2 = sc.nextDouble();
System.out.println("두 번째 원의 반지름을 입력하시오 >> ");
Double radius2 = sc.nextDouble();
Double dis = Math.sqrt((x1-x2)*(x1-x2)+(y1-y2)*(y1-y2));
if (dis < radius1+radius2)
System.out.println("두 원은 겹칩니다.");
else
System.out.println("두 원은 겹치지 않습니다.");
sc.close();
}
}
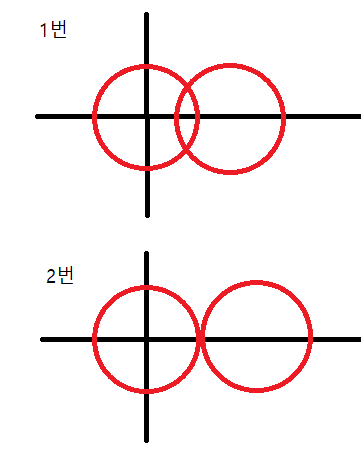
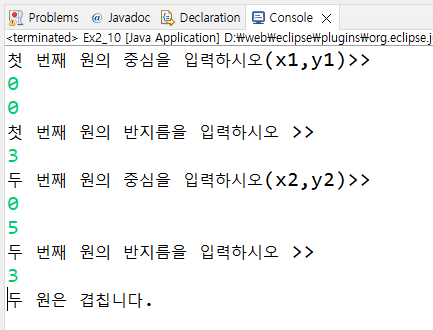
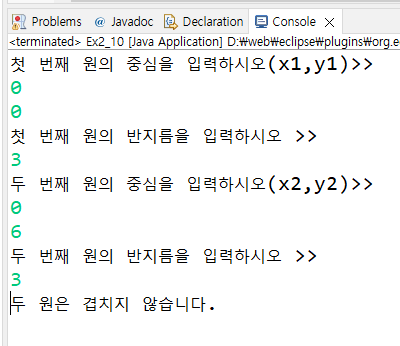
2장 실습문제 11번 : 숫자를 입력받아 3~5는 "봄", 6~8은 "여름", 9~11은 "가을", 12,1,2의 경우는 "겨울"을 그 외의 숫자를 입력한 경우 "잘못입력"을 출력하기
public class Ex2_11 {
//숫자를 입력받아 3~5는 "봄", 6~8은 "여름",
//9~11은 "가을", 12,1,2의 경우는 "겨울"을
//그 외의 숫자를 입력한 경우 "잘못입력"을 출력하기
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("월을 입력하시오 >> ");
int a = sc.nextInt();
switch (a) {
case 12:
case 1:
case 2:
System.out.println("겨울");
break;
case 3:
case 4:
case 5:
System.out.println("봄");
break;
case 6:
case 7:
case 8:
System.out.println("여름");
break;
case 9:
case 10:
case 11:
System.out.println("가을");
break;
default:
System.out.println("잘못입력");
}
}
}
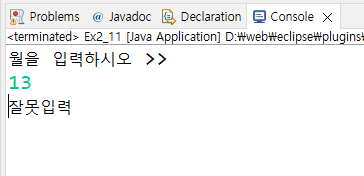
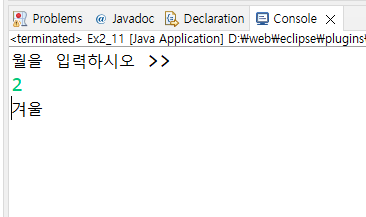
2장 실습문제 12번 : 사칙연산을 입력받아 계산하는 프로그램을 작성. 0으로 나누기 시 "0으로 나눌 수 없습니다."를 출력
public class Ex2_12 {
//사칙연산을 입력받아 계산하는 프로그램을 작성.
//0으로 나누기 시 "0으로 나눌 수 없습니다."를 출력
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Double a,b,c;
char mid;
int count=0;
String temp;
System.out.println("사칙연산을 입력하시오 >> ");
a = sc.nextDouble();
temp = sc.next();
b = sc.nextDouble();
mid = temp.charAt(0);
c=(double)0;
switch(mid) {
case '+':
c=a+b;
break;
case '-':
c=a-b;
break;
case '*':
c=a*b;
break;
case '/':
if (b==0)
count++;
else
c=a/b;
break;
}
if (count==0)
System.out.println(a+""+mid+""+b+"="+c);
else if (count==1)
System.out.println("0으로 나눌 수 없습니다.");
sc.close();
}
}
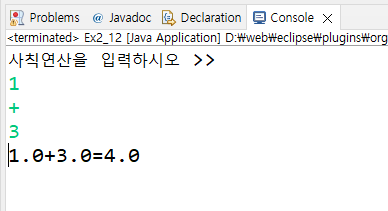
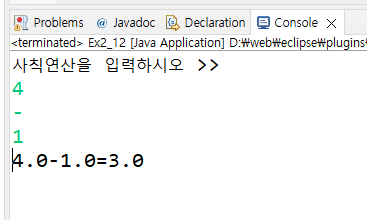
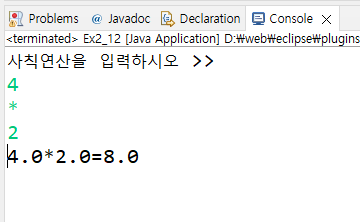
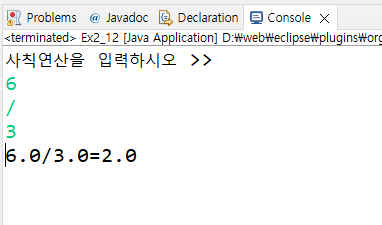
* temp.charAt(0);
charAt(0) 을 쓰면 temp의 첫번째(0번째)문자를 가져온다.
마찬가지로 charAt(4) 는 4번째 문자를 가져온다.
따라서 사칙연산으로 입력하는 문자를 가져와 mid에 저장하는 것
'개발자 세릴리 > JAVA' 카테고리의 다른 글
[JAVA] 명품자바프로그래밍 6장 실습 (0) | 2023.03.16 |
---|---|
[JAVA] 명품자바프로그래밍 5장 실습 (0) | 2023.03.13 |
[JAVA] 명품자바프로그래밍 4장 실습 (0) | 2023.02.28 |
[JAVA] 명품자바프로그래밍 3장 실습 (2) | 2023.02.25 |